useQueryClient is not working as expected
So before v10 I was simply using useQueryClient and had my
queryClient
strongly typed. Now I moved to v10 and for some reason, the documentation says that the queryClient was removed from the context so I should use the useQueryClient hook directly from @tanstack
but if I do so then the queryClient returned by that hook is not the queryClient trpc
is using, it's just empty. I made it work by using the trpc.useContext
hook coming from my client utils but I definitely think that the docs are a bit f*cked up.40 Replies
Code that used to work
How I made it work in v10
So I'm in v10
but the docs explicitly say that in v10 the queryClient was removed from the context and that we should use useQueryClient coming directly from the tanstack dependency
Migrate from v9 to v10 | tRPC
- tRPC v10 is ready for production and can safely be used today.
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
On my phone but to me that all looks correct, you’re supposed to use the proxy utils methods. If anything your usecase from v9 was ”incorrect”. The raw queryclient shouldnt be needed very often 🤔
Im also not sure why you’re setting the data on success instead of just invalidating the cache but thats a different issue
Well, it depends on the use case. If my app would be used by multiple users at the same time with the same source of truth, then yes, I need to be up to date with the server data. But if my data is only mutable by the current user, then there's no need to refetch the data if my API returns the mutated entity.
In any case, that's another issue. The app works, and yes, it makes way more sense to use the trpc context instead of the queryClient. I'm just surprised that the migration guide explicitly says otherwise. It's a bit confusing
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
Perfect then, thank u very much. Freakin love this library
Not sure I follow. We dont recommend using the raw queryclient do we? We just state in there that it has been removed so that people who were using the raw client from the trpc client knows they have to change it
So by this I understand that there's no access to the queryClient through the context anymore and that we should start using the queryClient directly from
@tanstack/react-query
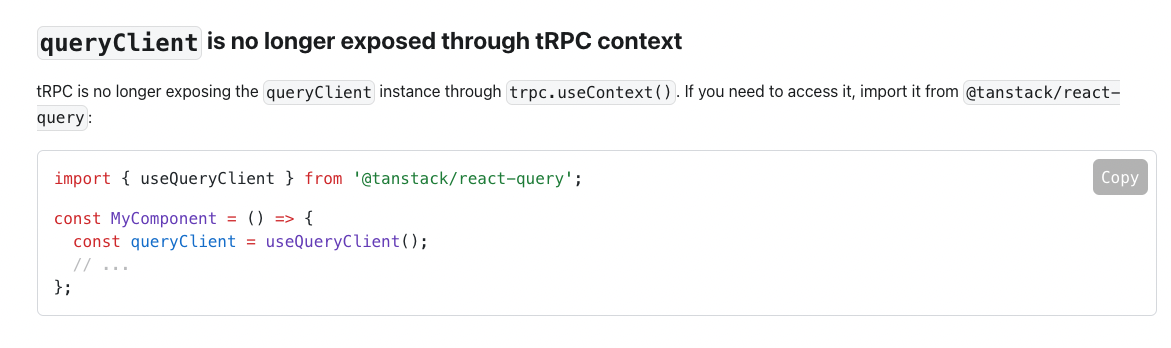
then maybe we should rephrase it
what it means is
the other methods are still there, just moved to the proxy
but this is (supposed) to point to the queryClient alone
fyi, the raw queryclient has always been un-typesafe
Yup, u're right, that's why to me using useContext is WAY better
I mean, I could use the queryClient for other non-server-related data, but I'd say its quite a bad practice. Now, having said that, the queryClient coming from useQueryClient will always be empty, so why would I use it?
there are things the trpc proxy methods doesn't support yet
see here https://github.com/trpc/trpc/issues/3012
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
i think Jon is working on that issue to implement more features on it
yes, but the queryClient coming from useQueryClient is a separate client, is not connected at all to trpc's...
yes it is, it's all coming from the same context-provider
useQueryClient
is pulling the qc from context, and your app is wrapped with a react-query context provider
if you're using next (withTRPC), we defined it for you: https://github.com/trpc/trpc/blob/e94acb19c440be7aedfde4b24207ea3f5657a986/packages/next/src/withTRPC.tsx#L132
you're not creating a new client when you do useQueryClient
if that's true, this should be true (let's assume the data is a primitive value for now)
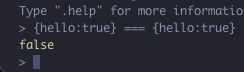
(let's assume the data is a primitive value for now)
oh sorry
no problem
gimme 2min i'll make a repro
yup
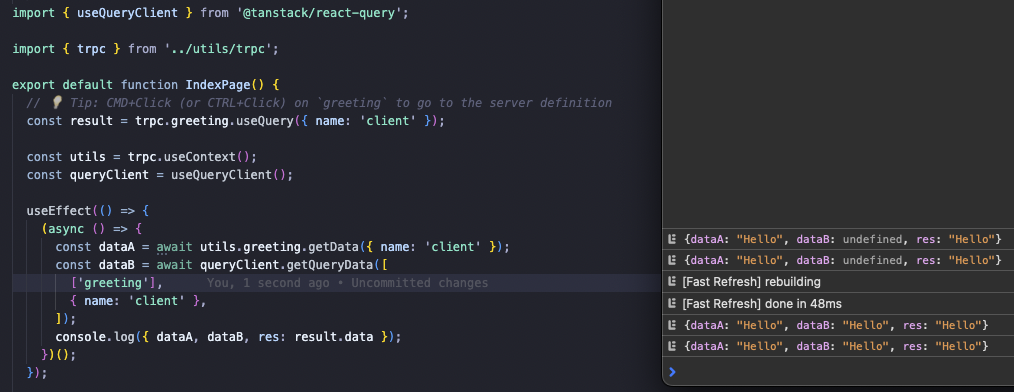
hmmm I defintely was not awaiting for the changes, maybe that's where I went wrong
i just did to make sure
I'll try real quick
thanks for the quick response in any case
works without too
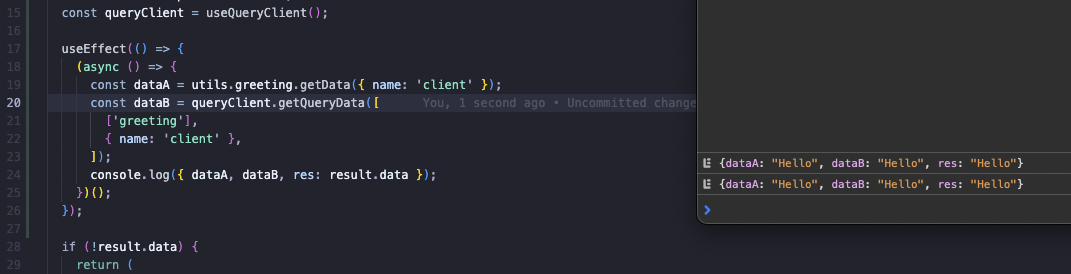
hmmm
I wonder where I f*cked it up then
hahaha
make sure you double check the querykey
the path should be its own array
I'll try real quick and be back
so like post.list =>
qc.getQueryData([["post", "list"]])
and if you'd have any input that would go after the path-array qc.getQueryData([["post", "byId"], { id: 1 }])
hence why its much easier to use the proxy 😉 post.list.getData()
doneDone, found my error, it was the nested array
Thank u so much, in any case I still will continue to use trpc's context for type safety
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
btw, I tried using the dev tools u have mentioned in your docs but they don't work very well. Are there any plans to have RQ dev tools for trpc? Or maybe even using the exact same ones as RQ does? If I'd known that the data was being persisted in that queryClient I think I might have caught the problem sooner
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
found the issue =>
(if you're using tRPC v10, install the @next version)
nice
sorry for the questions
I feel dumb as hell
anyway, thanks again 🫶Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
already made it work and is more than enough
thank u!