Looking to fix my tRPC implementation
Hi guys I am looking for some help implementing tRPC in my current project, I have 3 files that need to be reviewed I think I almost have it but for some reason I am getting some errors. My goal is to replace the restful API endpoints and instead use tRPC.
My first file is:
src/server/app.ts
Content:

15 Replies
Second file:
src/server/tRPC/index.ts
Content:
Third file: src/client/tRPC/index.ts
Content:
Whats my goal? I would like to get results using this query for example:
This is my result:You’ve added a prefix on the server but the errors don’t show the prefix
So you need the prefix on your client
Should be the same prefix @Nick Lucas ? or something different? Sorry I am new on this
It's just a HTTP URI
You wouldn't take out a rental agreement at 231 Spooner Street and then be surprised when all your stuff isn't in 234 Spooner Street. It's a different location
If you host tRPC at one URL, don't be surprised when a different URL returns a 404
Ok I have added this:
src/client/tRPC/index.ts
Result: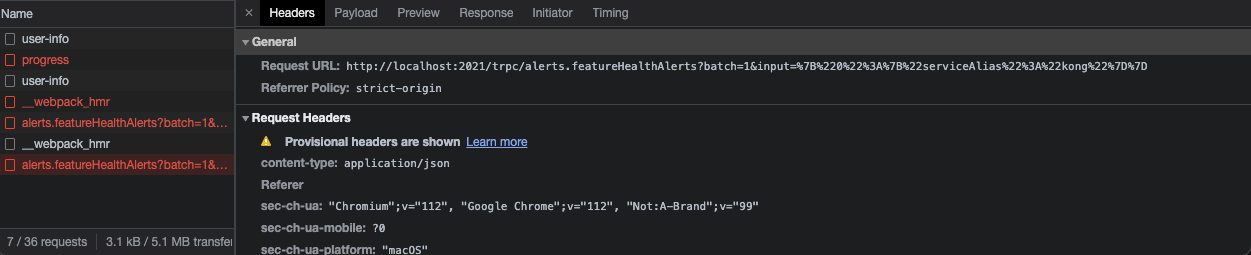
What's the actual error code now?
And is the port you've set actually correct? I don't see that in your API setup
I am getting this:

My app uses 3000
What port is the API on though?
the updated code is port 2021, the error says 4000. Something's wrong 😄
Yeah I updated it one sec
My app works on this port:
https://localhost.sparkcognition.com:3000/
I mean reactjs, so should I use this config:
This is where I am not sure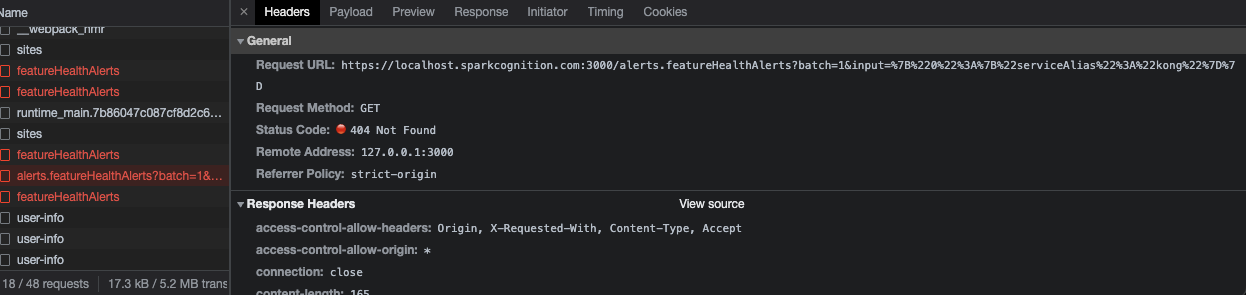
Hi @nlucas sorry for bothering you, please let me know when you have a few mins to help me with this, thanks
This strikes me as needing to go back to basics a little. Setting a URL on both sides isn’t that hard, but throwing tRPC and next into the mix isn’t helping you
Why not follow an Express tutorial and just try to get a simple rest endpoint going and a simple react spa connect to it?
Then you can start swapping out parts and add tRPC to the express API
I am following this guide but I am still getting the error: https://codesandbox.io/s/github/trpc/trpc/tree/main/examples/express-server
CodeSandbox
@examples/express-server - CodeSandbox
@examples/express-server using @trpc/client, @trpc/react-query, @trpc/server, express, zod
@Nick Lucas I managed to fix it, thanks so much for the help! it was related with the address:
Server
Client