[How To] Properly use trpc UseQuery based on currently selected item
I have a component with a video element and a flatlist. I want to utilize a trpc query to get the videoUri based on the currently selected item.
Here is my working component:
26 Replies
I can use this component in a screen and pass in a {data} prop to override the items and the videos it loads.
I can use a basic trpc query to fetch all posts from my 'wp_posts' table and load them into my component. The query looks like:
Now in my actual database the videoUri lives in another table 'wp_postmeta' and not in the same table that would have the "post_title". I understand I could modify the 'all' trpc query to add the videoUri from the 'postmeta' table into the one query. But this would add unnecessary time to loading the original flatlist which only needs the basic information like post_title.
I assume it is better to call a second query inside the Component that fetches the videoUri based on the currently selected item (currentItem.ID) via a second query function like so:
How would I properly call this second query that only fires once currentItem has a value? I tried placing it insdie a useEffect hook with a condition of [currentItem] but got an error. This is what i tried:
Some research tells me that useQuery is a hook and cannot be called inside useEffect. (https://stackoverflow.com/questions/70300864/how-to-use-usequery-with-useeffect)
i am also trying to use something like:
but have not been able to get it to work
This is the way to go
Solution
thank you!
I currently have:
and am getting error:
Add superjson
Data Transformers | tRPC
You are able to serialize the response data & input args. The transformers need to be added both to the server and the client.
it seems i already have that done?
https://github.com/launchthatbrand/t3-turbo-and-clerk/blob/lms/packages/api/src/trpc.ts
GitHub
t3-turbo-and-clerk/trpc.ts at lms · launchthatbrand/t3-turbo-and-cl...
Contribute to launchthatbrand/t3-turbo-and-clerk development by creating an account on GitHub.
Not on a laptop so can't browse the code very well
Is it a native BigInt?
If it's a custom thing you have to add your own serializer
Superjson handles BigInt well
It might also be that you're using some query cache lib where this error comes from
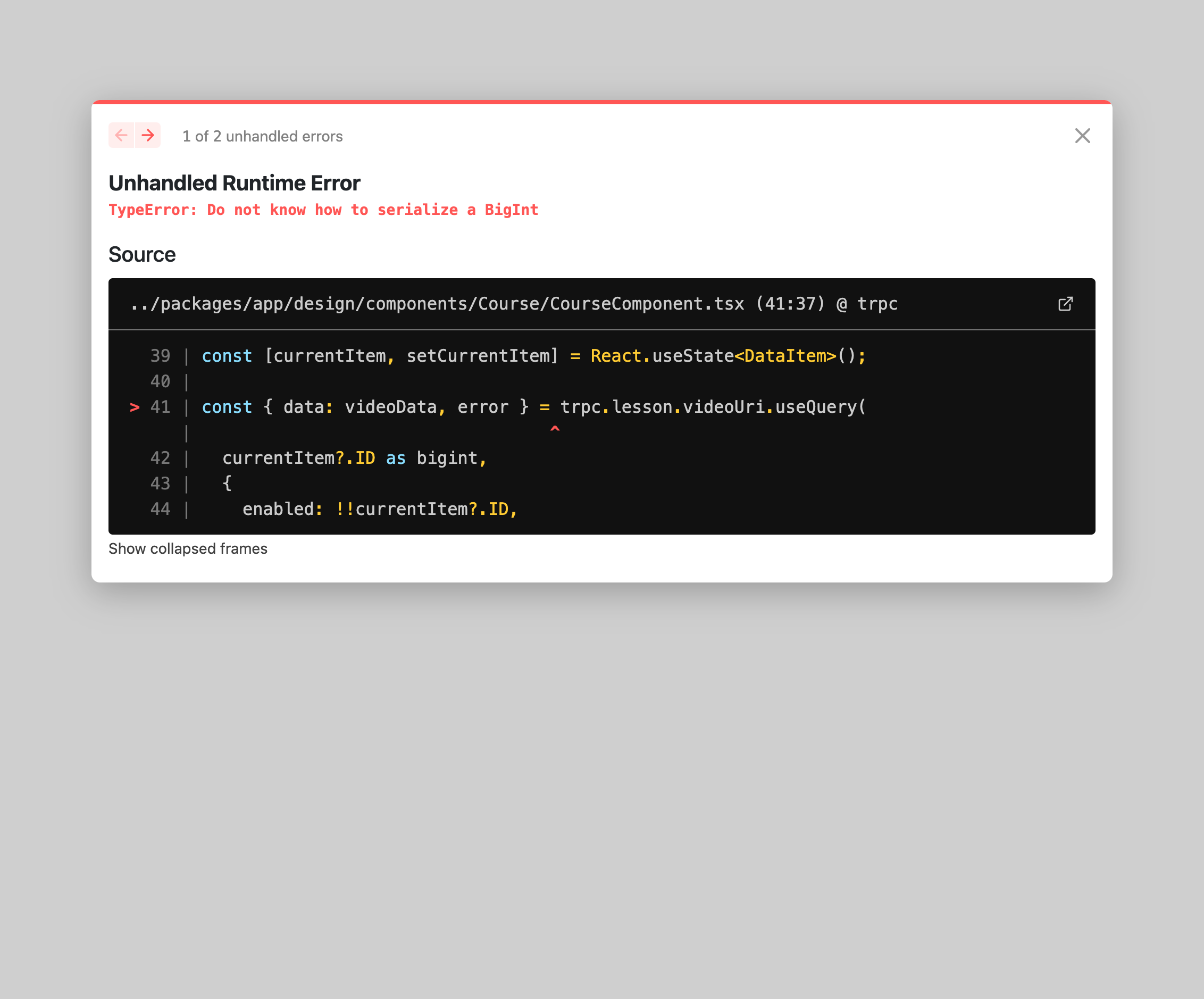
i am using trpc with prisma, and a wordpress database.
shema.prisma looks like:
lesson router looks like:
It looks right to me
If you want a quick ugly fix you can turn your BigInt into numbers or strings
Before returning them in the proc
Unsure why it's not working. I have a similar setup myself
in lesson router its ...input.(z.bigint()
and other places im using BigInt
does that matter?
if i try to use z.BigInt i get an error: "Property 'BigInt' does not exist on type"
Not really, if superjson is setup correctly it should work either way
Both for input and output
so where is the actual issue? in the trpc or prisma part?
since it goes trpc->prisma->db
if i change lessonRouter to:
so number rather than bigint, and then change the videoUri query with a hardcoded number I can get the proper videoUri in a console.log on the screen
so i have half the issue fixed, which was triggering the query based on the currently selected item. Now I seem to just be having an issue with bigint
trpc does a
JSON.stringify(xxx)
of the data sent and received
that will fail if xxx
contains anything that isn't standard json
if you use a data transformer it'll do JSON.stringify(transformer.serialize(xxx))
hmmm...ill look into making sure superjson is set up peoperly then, but its using the default setup for packages/api from t3-turbo:
I have "superjson": "^1.9.1", in package.json
its added to packages/api/src/trpc.ts
doing some tests now to ensure it's not on our end
im using "@trpc/client": "^10.1.0",
"@trpc/server": "^10.1.0",
yeah it's not on our end, just did a test
try upgrading trpc and superjson
trpc will give you type errors in later versions if you have setup the client wrong too
misconfig of transformers was so common so we made it into a type error if you did
should i use latest or a specifc version?
10.27.1?
latest
ok i changed all packages to ^10.27.1"
ran pnpm i
changed lesson router back to z.bigint
i changed the component query to what you suggested:
i am getting inline error:
and console error:
if I change component query to:
the inline error goes away but i am still getting the serialization console error
if i change to:
I get inline error:
as an update I added:
to the top of my coursecomponent file and it seems to have fixed the error
Reference: https://github.com/prisma/studio/issues/614#issuecomment-1186637811
Might be that react native doesn't support BigInt too
i accepted a solution to my og problem that this thread was titled after. I am still trying to address the issue with BigInts