How to retrieve and receive Bigint data to/from TRPC procedure
Node:
v16.15.1
I'm trying to return an object which contains an amount
property from one of my TRPC procedures... This property should retrieve a bigint
. Unfortunately, I'm receiving error:
TypeError: Do not know how to serialize a BigInt
When doing this. I've already configured superjson
Data transformer in my TRPC server instance creation and in client's createTRPCNext
's response, but this didn't solve the problem.
What is the most appropriate way of receiving and returning bigint
properties to/from my TRPC procedures inside my Next.js application?
I appreciate any help, thanks! 🙏🏼
These are my packages versions:
"@trpc/client": "10.14.1",
"@trpc/next": "10.14.1",
"@trpc/react-query": "10.14.1",
"@trpc/server": "10.14.1",
"next": "13.2.3",
"superjson": "1.12.3"7 Replies
You probably just need to register the type with superjson
It’s in their docs how to do this
Hey @Nick Lucas ! I appreciate your quick response, I'll take a look on this now and let you know in what it results 😉
@Nick Lucas
Adding the following code wasn't enough for getting rid of the error:
TypeError: Do not know how to serialize a BigInt
registerCustom<bigint, string>(
{
isApplicable: (v): v is bigint => typeof v === "bigint",
serialize: (v) => v.toString(),
deserialize: (v) => BigInt(v),
},
"bigint",
);
Do you have any other suggestion on how to fix this issue?
I'm send attached to this message my TRPC server file + TRPC client file so you can have more visibility of how I'm implementing things here 👍
You’ll need to add it on both client and api if you haven’t, otherwise I’d check their GitHub issues
I've extracted the registration of this custom type to a file which is being imported in both client and server TRPC configuration files, but the error below persists:
TypeError: Do not know how to serialize a BigInt
I wonder if this is related to TRPC procedures config, to SuperJson lib (which should give support to BigInt without the need of registering new custom serialize/deserialize function for it, as mentioned in their docs: https://github.com/blitz-js/superjson#examples-3) or to Next.js
Neither SuperJson, TRPC nor Next.js Github issues contain related issues, so I'm finding myself completely stuck in this limitation now 😮💨
GitHub
GitHub - blitz-js/superjson: Safely serialize JavaScript expression...
Safely serialize JavaScript expressions to a superset of JSON, which includes Dates, BigInts, and more. - GitHub - blitz-js/superjson: Safely serialize JavaScript expressions to a superset of JSON,...
The workaround is to extend BigInt by modifying the prototype
Create a
bigintPatch.ts
file and paste this code then import it like import "bigintPatch.ts"
on the page where you’re calling the query/mutationHi @NEO
I've added something very similar (https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/BigInt#use_within_json) to the code snippet you've shared and the issue was fixed! 🥳
Thanks so much for your help @nlucas and @NEO, I really appreciate it! 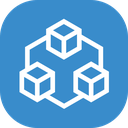
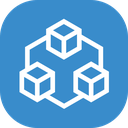
BigInt - JavaScript | MDN
BigInt values represent numeric values which are too large to be represented by the number primitive.