problem with useContext (likely easy fix)
could someone please explain what im doing wrong thats giving me this error? cheers
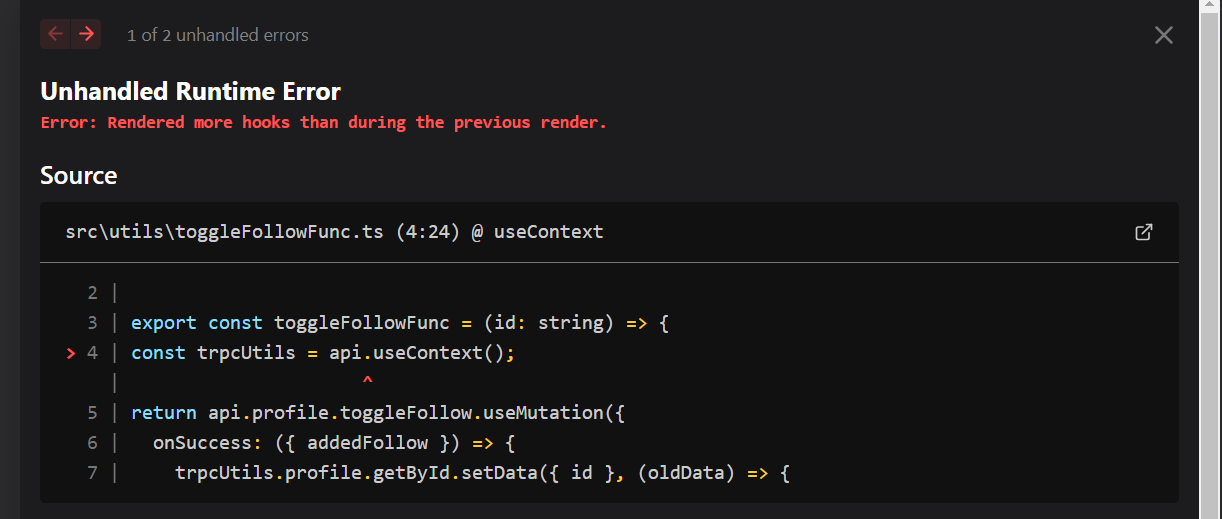
2 Replies
it was working before when you could just follow one person on their profile but now that I am trying to add the option to follow their followers as well it breaks
It's actually pretty simple to fix: just move trpcUtils declaration outside of a function. You should probalby get the desired data inside a component and pass it as a prop.