tRPC querying data via function not working
In the below code,
fetchSocialMediaData
is logging themeId
but, not response
, getting error as mentioned in the screenshot attached. Is there anything I missed from docs? Correct me wherever I am wrong.
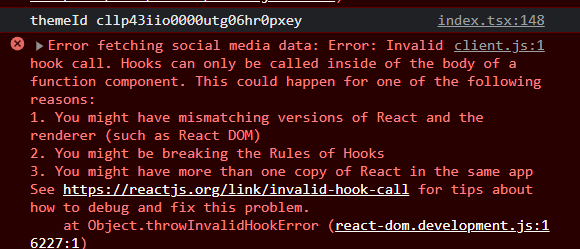
1 Reply
The error is pretty self explanatory. You are calling a hook somewhere that is not a hook or component. I cannot see from your code example what the problem is but if you have your code snippet running in a function try rename it as useFetchSocialData or make sure the code is ran as part of the top level of a component. You can also not call a hook conditionally (inside an if) so keep that in mind as well. Also your fetch functions should be probably inside the useEffects or using a useCallback if they are called from other places and your useEffects currently have missing dependencies on the dependency array. They are now recreated on every rerender.