Unable to get mutation to trigger subscription because EventEmitter not being shared
Hey folks,
Been struggling with this for a few hours now hopelessly and trying random things - read all related posts in this forum, on github issues, and stackoverflow - and still don't understand what is going on.
I have a next app with a custom HTTP server and using tRPC. WSLink etc is all fine - i'm doing everything the proper way.
I have a router with these two functions:
I've added a bunch of console logs to help explain.
So, in my logs I see that when the app loads up I get a WS Connection to the server.
On the client side, I have
trpc.chat.onNewMessage.useSubscription
which console logs {names: []}
first and then {names: ['onNewMessage']}
as it should based on the code for the router.
But, when I send a message, the mutation fails to trigger the subscription because it logs {namesSendMsg: []}
i.e. for some reason it does;n't recognize the attached listener
I thought it was because maybe somehow multiple EventEmitter
instances are being created due to HMR - so I did a workaround similar to what we do with Prisma for next dev mode:
src/eventEmitter.ts
:
this did not help either. using this wsee
everywhere doesn't change anything. im lost and have no idea WHY there are supposedly two different event emitter instances being used here?2 Replies
my terminal output basically looks like this:
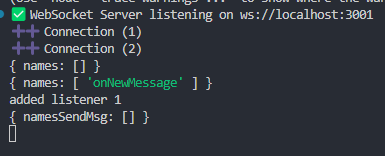
FWIW - i cloned the example websockets repo and that works just fine. so something somewhere is wrong, but i have absolutely no idea what. any hints would be great.
my tRPC is initialized as such:
Sorry to tag but cc @Alex / KATT š± - another person mentioned this issue in another thread with no response and I feel like Iāve tried everything short of digging into Trpc codebase and trying to figure out whatās going on
Edit - the other issue is https://discord.com/channels/867764511159091230/1125838439393271958
I also tested with creating a simple subscription route that just sets an interval and emits random data to the frontend - this works just fine
The problem is simply that two different instances of the event emitter seem to be used in the mutation vs the subscription, causing the mutation route to believe thereās no attached listeners when there are
And I have no idea why there are two different instances :/