Can set cookie with trpc?
I tried to create a full authentication system in trpc using jwt and refresh token
I find that is not quite okay to create authentication with trpc
Can you suggest me a better way how I can achieve authentication in trpc project ?
21 Replies
Why do you find it not okay to create auth with tRPC?
The most simple solution would be to implement a route with which you can login, which then sets a JWT cookie. And then create a protected procedure that checks someone’s JWT,
Most projects will be using auth frameworks like LuciaAuth, NextAuth or Clerk etc.
I can not send cookie via trpc, I tried a lot(if I use with express)
I know with clerk to do auth, I am just curious to do it with jwt
You can set cookies in Express using res.cookies
So as long as you give tRPC access to the res object, you can send cookies
Otherwise you might want to send the JWT in the body of your response and send it as a header with every request
in my browser in cookie i can not see token
@BeBoRE
You are creating a query, this should be a mutation
If you changed that and it still doesn't work, see if you see the set-cookie header in your response headers
okay, i will change it to mutation
i let you know if it works
What is the raw response for the happy flow?
?
@BeBoRE
i modigy tu mutation

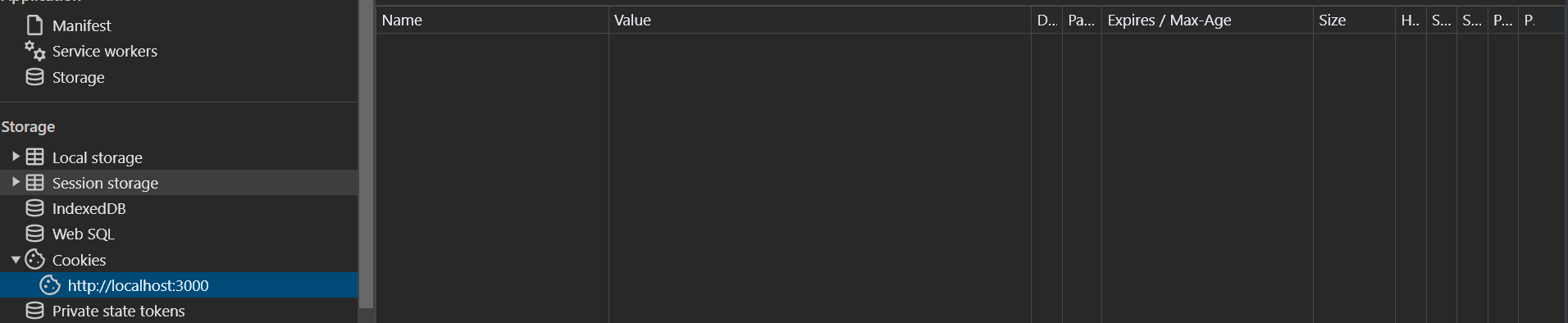
nothing
Using HTTP cookies
SameSite=None only works in a secure context with HTTPS, you probably want to set that attribute to lax which is the default
MDN Web Docs
Using HTTP cookies - HTTP | MDN
An HTTP cookie (web cookie, browser cookie) is a small piece of data that a server sends to a user's web browser.
The browser may store the cookie and send it back to the same server with later requests.
Typically, an HTTP cookie is used to tell if two requests come from the same browser—keeping a user logged in, for example. It remembers st...
Ok
Still now idea how to solve it
Only idea that I used is when I send token on client, I use some js cookie or smt like that and from there I save it to cookie
Pretty sure if you remove
sameSite: 'None'
it should set the cookie correctly, also in the screenshot you send it looks like if you use my cookie
as the cookie name, I don't believe you can have spaces in your cookieOk, I will check it
@BeBoRE

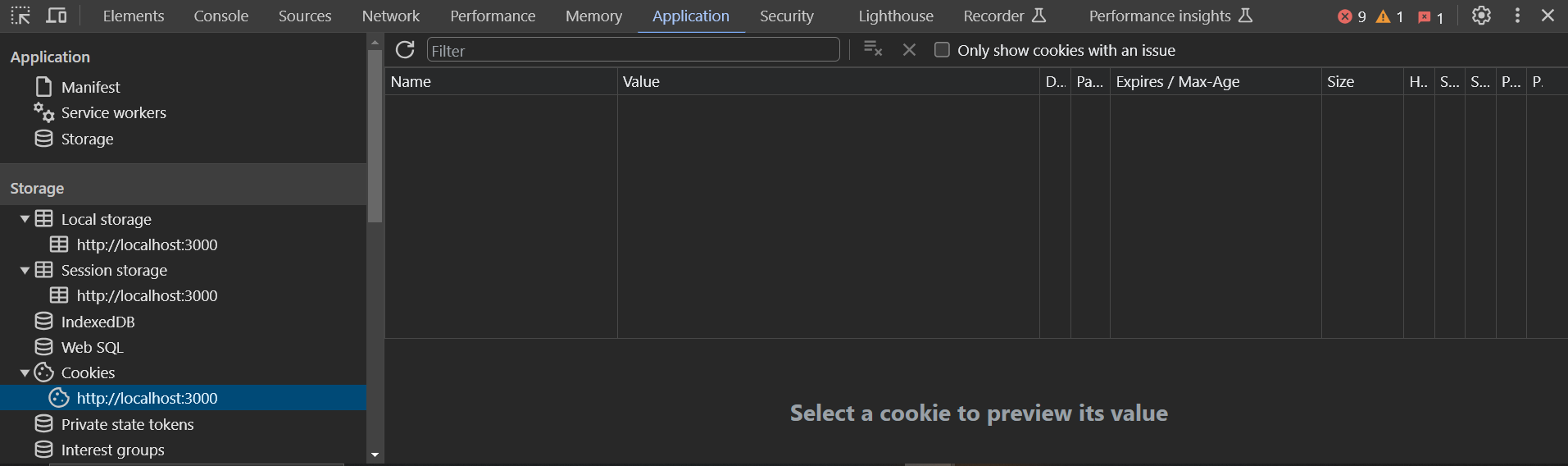
i do not understand where i do wrong
nvm, i solve it
What was the issue?
i forgot to add when i create express be
🙂
i add that and now works fine
Ah the empty cors made it so that the cookie wasn’t being send with, ngl I have never understood CORS
:)))
3 days for a fu….ing CORS