Beginner Client Problems
Node 21, npm 10.2.4, tRPC 10, from a create-t3-app run yesterday.
I've got a zod validator create by drizzle-zod, for inserting into a table. There is a
.omit()
call to remove fields not allowed to be set by my user. I'm using this in a publicProcedure as the argument to .input(TableValidator)
, and there's a .mutation()
that inserts the data into the database.
I have also used a z.infer<typeof TableValidator>
to get a type for this data, which I'm using on the client to coerce the single data structure I'm using as test data to that type, to use as an argument to the .useMutation()
call.
My editor thinks that the data structure type does not match the expected input type of the .useMutation()
. The error message reads:
Type { command: "add" | "remove"; argument: string }' has no properties in common with type 'UseTRPCMutationOptions<{ command: "add" | "remove"; argument string; },TR{CCLientErrorLike<BuildProcedure<"mutation", { _config: RootConfig<{ ctx { db: BetterSQLite3Database<...>; }; meta: object; errorShape { ...; }; transfor...'
and the parameter type.
How do I go about finding what I have failed to understand?4 Replies
Further details:
schema.ts:
usbDeviceControl.ts:
root.ts:
Client in addWorkItem.ts, to as a standalone client, to add items to test:
addWorkItem.ts will be run by tsx, in the dev phase.
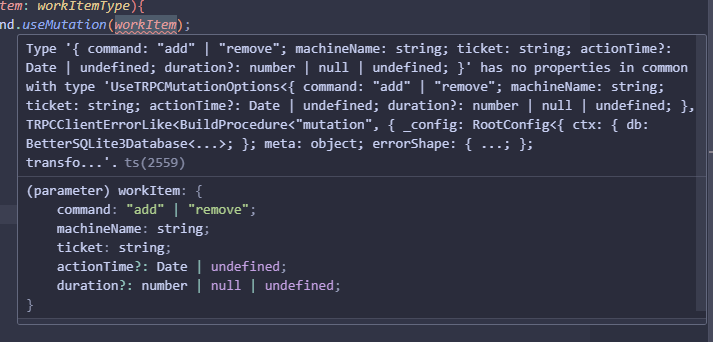
You need to have a read of the react query docs because that’s not how you use useMutation
Solution
Our docs also will have examples
aha. The
createTRPCProxyClient
call was my stumbling block. That and having been redirected to the v11 docs without realizing it.
Thank you for the help.