My TRPC hooks are getting typed as `any`
I'm using a Typescript + Express backend (Node.js, version 18), and a React Native (Typescript) frontend. For some reason by TRPC tanstack-query hooks don't seem to be typing things correctly. I am trying to understand why.
I have a monorepo setup with a file structure like this:
root
-frontend
-backend
So the frontend and backend files can import from each other, and everything lives under one
.git
in the root.
In my backend, I create a router and export the type:
Here is an example typing I get when I hover over a procedure in one of my subrouters. I don't return any
in any of my procedures.
In my React Native app, I import the type:
Then in my App.tsx
, I create the trpcClient
:
Now when I use a hook:
My data
gets typed as any
, what am I doing wrong in my setup?5 Replies
I've managed to distill the issue even further:
When I make this procedure on the backend:
Then my frontend hook
data
field gets typed correctly.
But if I use a prisma query:
My frontend hook data
field gets typed as any
. Why is this happening? My prisma query is certainly not returning type any
.Here is an image of the return type of my prisma query.
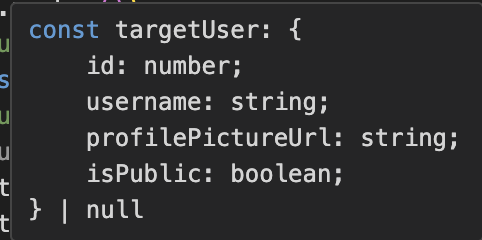
What's even weirder is if I cast the return type:
Then my frontend
data
gets typed correctly as number | undefined
. But if I remove the cast, turning it into the typing as pictured above, then the frontend starts getting any
.I got it fixed by following this four year old twitter thread: https://x.com/colinhacks/status/1298030688063561728?lang=en
Colin McDonnell (@colinhacks) on X
"nohoist" is literally not documented anywhere except in the blog post where it was introduced
https://t.co/SuobFbSq4z
Twitter
Is there a reason this isn't listed in the docs anywhere (or did I miss it?). It seems like it should be a pretty common issue given how prevalent prisma is. I get it isn't an issue with TRPC per se, but it does seem like it could have a useful place here. I'd be happy to make a docs contribution.