I commented my query out, but i still notice request are still been made. this is what my code look
I keep getting api request to backend continously even without making api request. my api client
7 Replies
@Sigo sorry what request is being made here? is it the retrieval of supabase credentials occurring multiple times?
It trys to make request to the query which i already commented out and it keeps retrying. i think it has to do with caching of request i guess
I turned off retries but still getting same issues
test
this is how am making the query
@BeastFox
the network tab
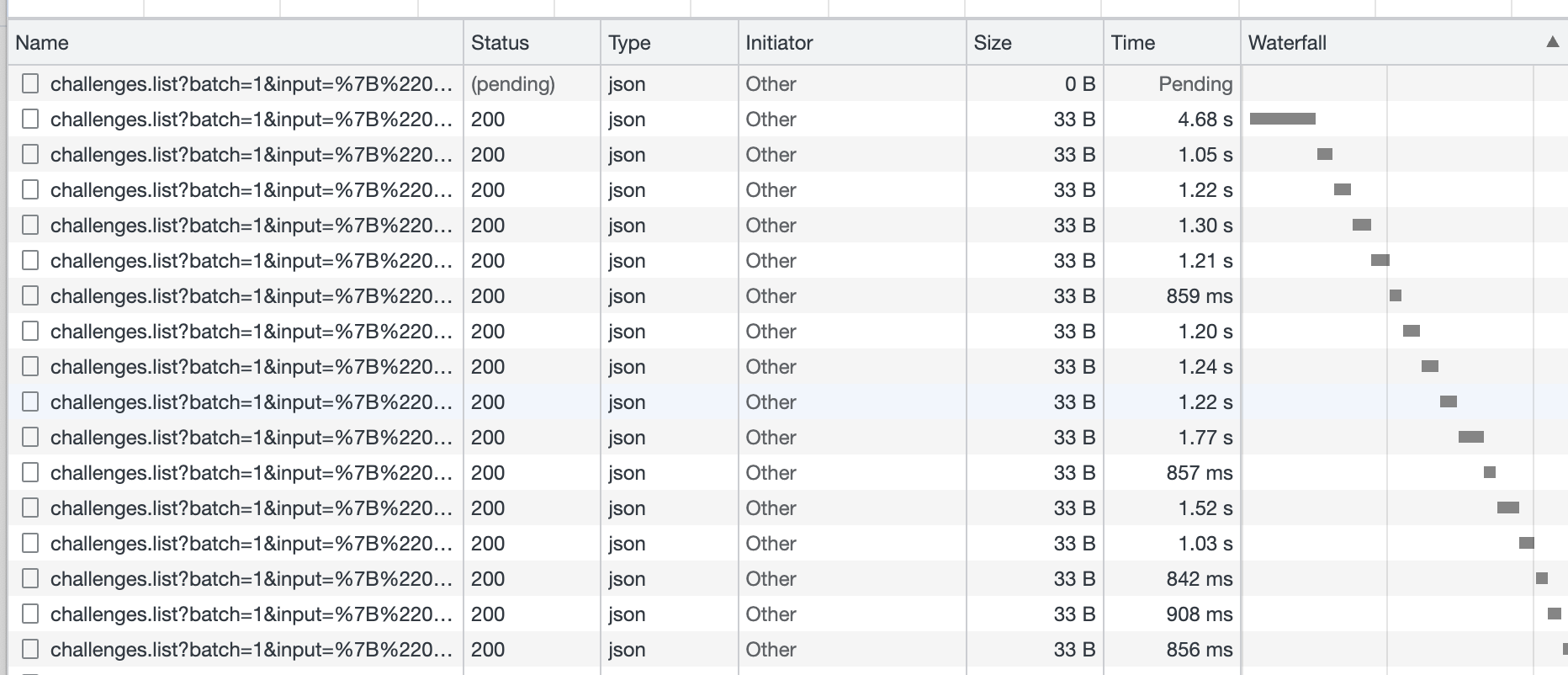
@Sigo what happens if you hard code the values in? Something like the following:
Just to try and eliminate any external state variables first.
same thing also happening
My only other idea is that it's something to do with your TRPCProvider, if getHeaders is being called multiple times that would suggest either a higher level context is recalculating it I think, or that something is happening in your useSupabaseClient.
I can't be too sure, so someone else would have to chime in if they have other advice. But my recommendation in situations like this would be to try and strip back your code as much as possible. So you have the most basic implementation created where you successfully have it so that only one request occurs.
https://trpc.io/docs/client/react/setup
Basically following the above here ^ (But hard coding the api.challenges.list.useQuery input etc..)
Then, begin slowly adding things back until you can see where multiple requests occur.
Set up the React Query Integration | tRPC
How to use and setup tRPC in React
i will try that
thanks