Cannot read __untypedClient from undefined error when try to update to latest 11.rc### versions
We are using TRPC with react and we got everything running with the latest versions from tanstack/react-query, but every time I try to update our trpc packages:
"@trpc/client": "11.0.0-rc.660",
"@trpc/react-query": "11.0.0-rc.660",
"@trpc/server": "11.0.0-rc.660",
To their latest builds we get an uncaught exception on the trpc.Provider component that it cannot read __untypedClient from undefined. I have tried to Google and check GitHub issues if someone faced the same or not, but to no avail. Is there someone out there who could point me some things to fix, check or otherwise to get me unstuck from the 660 builds?
24 Replies
That actually sounds like you're missing a provider or haven't created the client/provider properly
Can you share a repro?
I would not know where to start to get even something remotely close to our setup in StackBlitz or similar... but i will share some code snippets i think are relevant maybe it is something very obvious:
We have a file named trpc.ts that contains the following:
then we have a QueryProvider.tsx file that contains
the @/utils function createQueryClient is defined as:
and the useTRPCClientLinks file imported in the QueryProvider.tsx file is like this:
My assumption is that it fails here:
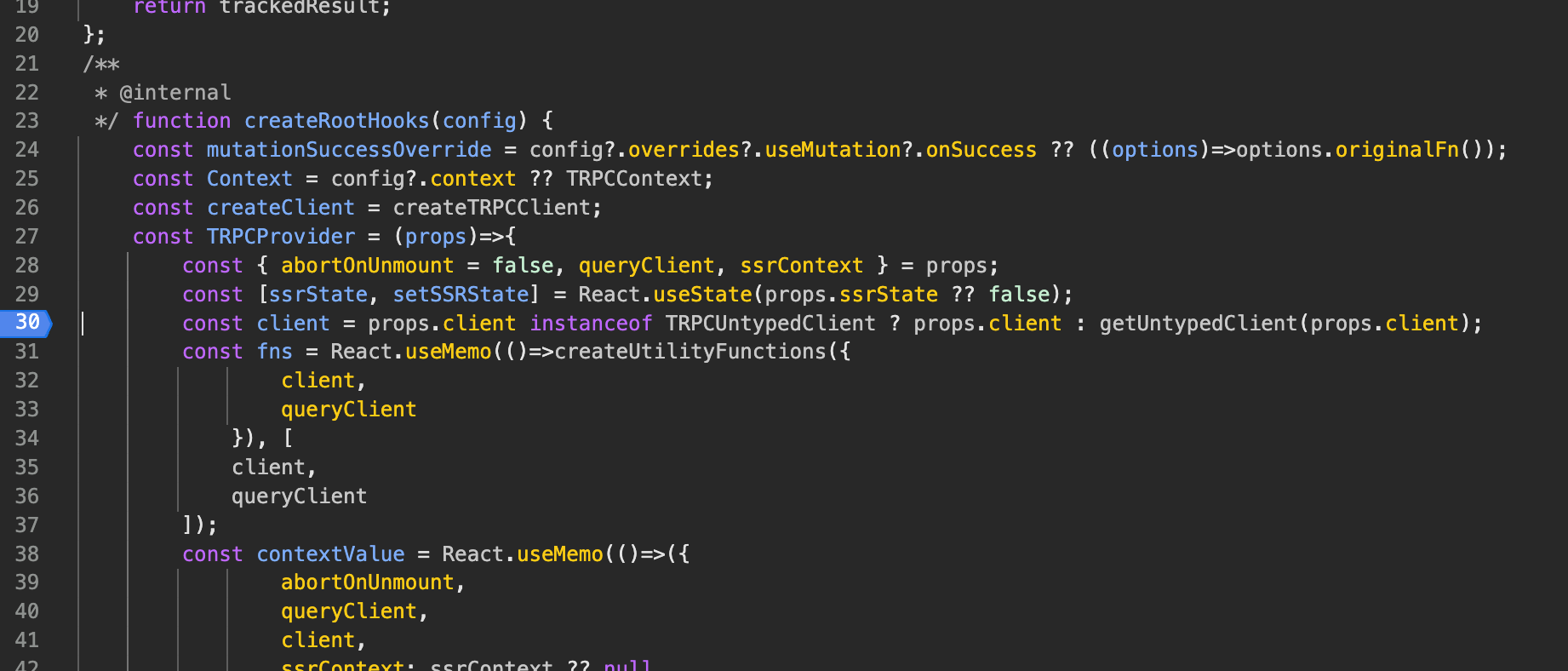
all the code works on version
11.0.0-rc.660
but when updating to the latest 11.0.0-rc.688
it fails with the error:
Hm @Alex / KATT π± did we change anything which could affect this? Iβm less familiar in this area
we did something here to fix another inverse issue
could you make an issue on github @wleistra ?
think there's no need for a full reproduction, just insert the info from above
Ofcourse I can!
GitHub
Cannot read properties of undefined (reading '__untypedClient') on ...
Provide environment information Works on "@trpc/client": "11.0.0-rc.660", "@trpc/react-query": "11.0.0-rc.660", "@trpc/server": "11.0.0-rc.660...
do you have a bigger stack trace than this?
especially with sourcemap defined
do you know which function that is used when this happens?
did you update all of
@trpc/*
deps? are the version numbers matching across client/react-query etc?yes all version numbers are the same as I do yarn upgrade-interactive on my workspace
as it happens on the trpc.Provider which is high up on the hierarchy, i'm not sure what you mean with function... The code we have in place to create the client and props for the provider are the ones I added to the issue
On Monday, when i return to work I will see if i can get you a better stack trace. Any tips or preferences on how the stack trace must be generated/copied/created
If I'm the only one having this issue, is there something we do in our code snippets that makes this happen and that we can do another way to make it actually work?
I believe it's a bug but I don't know what call triggered it
I can't reproduce it in any of the example projects we have
I will try to dive deeper into it on Monday
my best guess right now is that you have multiple
@trpc/client
installed across your monorepo and that they are not hoistedI do have some package json files with the caret and the version and some without the caret... So I will check the yarn.lock file and put everything to the non caret version notation to ensure the same versions and let's see if that resolves it. I find it a bit strange though as all the trpc packages were updated to their latest versions so there should not be version mismatches
Ok so removed all the carets and installed latest version rc.700 and checked the yarn lock file which shows a single version to be used throughout the whole workspace
After some digging i found out that trpc is initialized as undefined
results in
Uncaught ReferenceError: trpc is not defined
When trying to debug into the createTRPCReact function the hooks seem to be created (at least nothing suspicious there AFAIK) but proxx is resolved to
we use React 18.3.1 and typescript 5.7.3, build with webpack
I guess my debug skills are not great, but for all i can see the createTRPCReact inner workings seem all broken when watching variables most of it is undefined. But then again, this is my first time trying to debug this deep π
full stack trace as presented on console
you're sure this is the culprit that causes the error? "trpc is not defined" is not the same as "Cannot read properties of undefined (reading '__untypedClient')"
it is currently my understanding that when watching the trpc exported from trpc.ts file it seems be undefined... But i do agree it is suspicious because you need to call the trpc.createClient() and it doesn't yell there =/
It could be undefined because it throws an uncaught exception and the error boundary is higher up so it unmounts it, causing it to be undefined... I guess I just have no idea how to check what is interesting for you.
could you try with version
11.0.0-alpha-tmp-issues-6374.694
on all the trpc packages?
did some random fumbling around in a PR that might or might not fix it lol
very hard when i can't reproduce itWill try it tomorrow first thing in the morning
Unfortunately it still doesn't work on that version, but the error message has changed to
It truly seems that i don't get a client defined... maybe my screen recording will help you with some more info
i am about 90% sure that you have duplicate installs of trpc then
i think you have one package reading from e.g.
/packages/backend/node_modules/@trpc/client
and another reading from e.g.
/apps/web/node_modules/@trpc/client
whereas both should be reading from
/node_modules/@trpc/client
but we should fix it regardless
i have some ideas, might send you another version to try later today
could you try 11.0.0-alpha-tmp-issues-6374.697
?will do
i checked my monorepo. We use Yarn v4 btw, and there is only 1 node_modules folder in the whole repo and i did a search on "@trpc/react-query", "@trpc/client", and "@trpc/server". and all three have the same value
11.0.0-alpha-tmp-issues-6374.697
Also my yarn.lock file has no other resolutions or references to any other versions
i found one difference but it has a caret so it shouldn't matter and it is on the API side
could this be the culprit?
the exact version the error occurred first was 11.0.0-rc.677, the rc.666 version still workedand from what I can tell is that the following line
returns like depicted on the attached image.
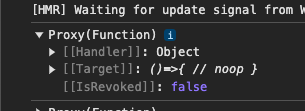
the console.loggin trpc.createClient
returns
then console.logging the full createClient call as
trpc.createClient({links})
returns
@Alex / KATT π± I got a similar problem, but I am running vite, so the stack trace is slightly different
but for me it starts with version rc.795
rc.791 works fine
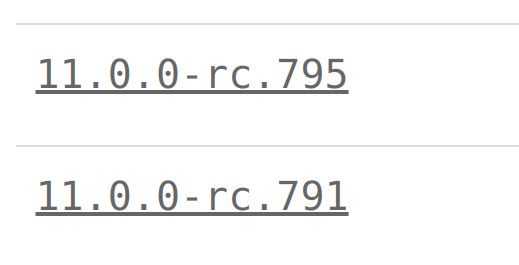