7 Replies
with the help of doc I came up with:
in my router
in my react app
in my my react component
Help is greatly appreciated!
I'm getting :
access-control-allow-origin:
*
connection:
keep-alive
content-type:
application/json
date:
Tue, 14 Jan 2025 16:21:09 GMT
keep-alive:
timeout=5
transfer-encoding:
chunked
vary:
trpc-accept
x-powered-by:
Express
response headers from my backend and
here is the error:
EventSource's response has a MIME type ("application/json") that is not "text/event-stream". Aborting the connection.
How do I make sure the backend nestjsTRPC put that header accordingly ?
I've tried to put @Subscription({ input?: ZodSchema, output?: ZodSchema })
But I can't seem to import that decorator
and it seems it's not present
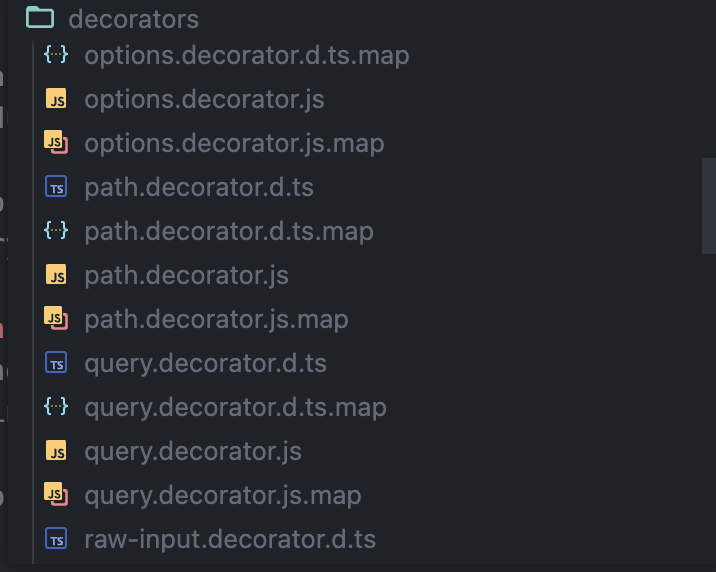
why is it not in the package?
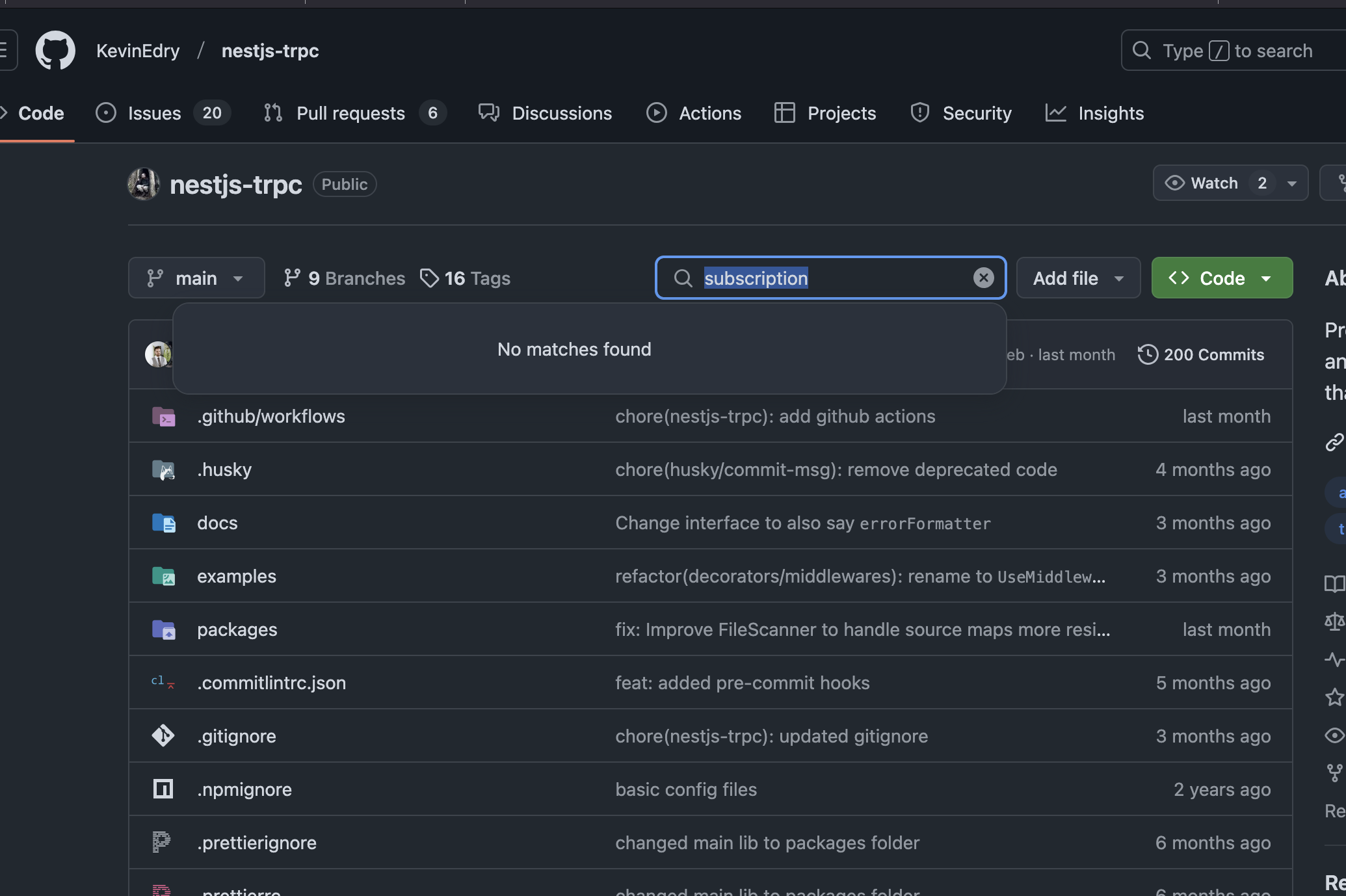
NestJS-tRPC: Bringing type-safety to NestJS
NestJS-tRPC Documentation - Routers
NestJS tRPC is a library designed to integrate the capabilities of tRPC into the NestJS framework. It aims to provide native support for decorators and implement an opinionated approach that aligns with NestJS conventions.
@julius Could you give me a hand ?
#🐯-nestjs-trpc for nestjs questsions
thanks!