Forward NextJs Request to TRPC Server
Hey everyone! I need some help with my project setup. Here's my current situation:
My Setup:
* I have a NextJS 14 project (which is both client-side and server-side)
* I also have a separate ExpressJS tRPC server
What I Want to Achieve:
I want both the client-side and server-side of my NextJS app to communicate with the tRPC server. However, I have a specific requirement for the client-side:
1. The client-side of NextJS should first talk to a NextJS route handler
2. Then, this route handler should make the call to the tRPC server
My Issue:
I'm not sure how to set this up correctly. I need help figuring out:
1. How to implement the NextJS route handler to act as a middleman
2. Best practices for this kind of setup?
Has anyone done something similar or have any ideas on how to approach this? Any tips, code snippets, or resources would be greatly appreciated!
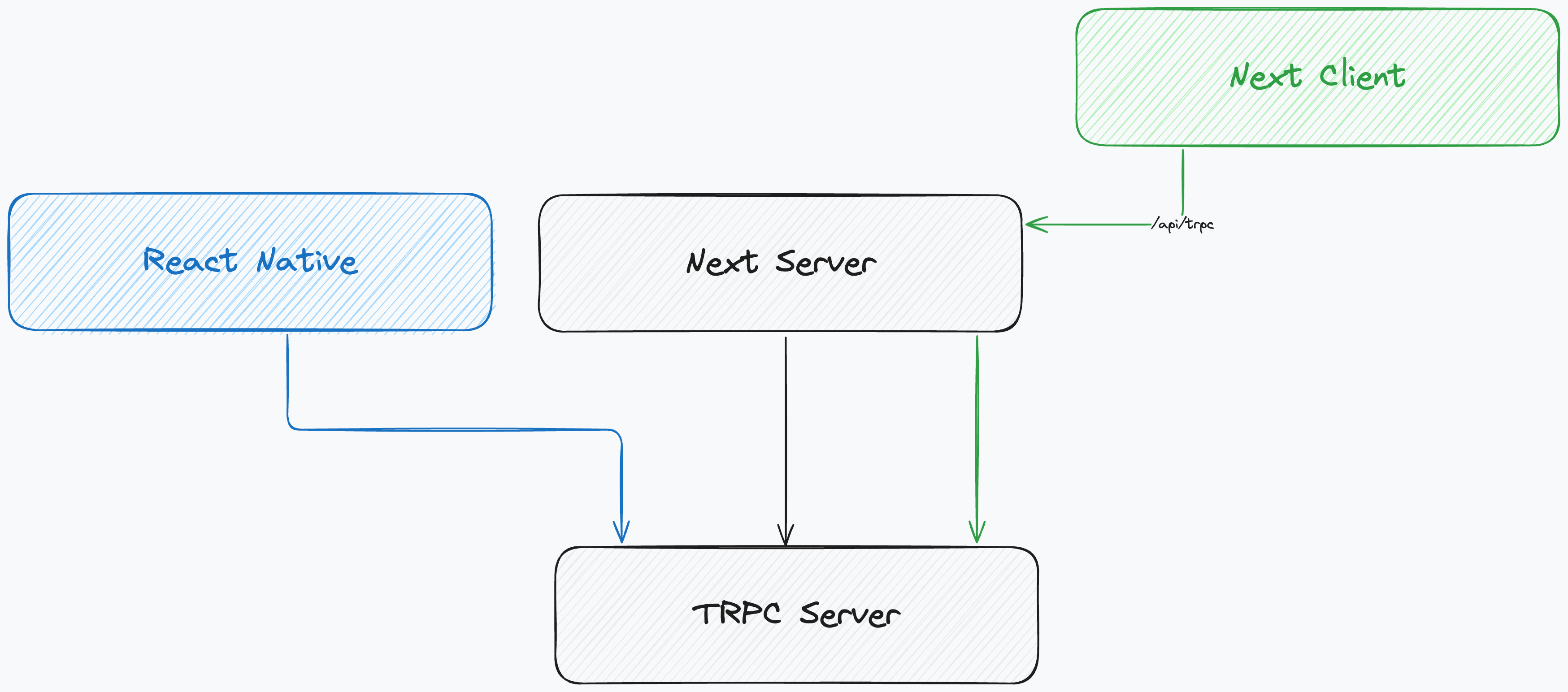
23 Replies
Hey, i’ll give my two cents but others might have better ideas.
Any chance you could give some context to why you want to split the servers up firstly?
Katt spoke about it somewhere a while ago, but if these are completely separate servers and not running off of the same service you’ll need to go over network for communication and look at the vanilla client https://trpc.io/docs/client/vanilla/setup. So you’d use that for your Next -> tRPC integration where Next calls your tRPC service.
The tricky problem to solve would be type definitions, to be honest Im not quite sure how best you’d achieve this. My only recommendation is that you pass the tRPC type definitions to your NextJS client additionally and then use things like RouterOutput/RouterInput
https://trpc.io/docs/client/vanilla/infer-types
which would allow you to still keep your data typed whilst still communicating first via the Next Server (you’d have to setup endpoint management etc yourself). But I don’t think it’ll be possible to get the full type definitions if you aren’t communicating directly between tRPC.
Unless you are basically mirroring endpoints between the next server and tRPC server it might be a bit tough to get the full feature set
Inferring Types | tRPC
It is often useful to access the types of your API within your clients. For this purpose, you are able to infer the types contained in your AppRouter.
About the context to why I want to split the server.
First of all all of the code sit in a Turbo monorepo.
we have a react-native app, and a nextjs web-app that both need to talk to the TRPC server for the common logic. as of today i have my TRPC server on NextJs and React Native is talking to next server with TRPC.
The problem we have is that each time i need to update some TRPC procedure I need to deploy and release NextJs as well, which is not favorable for us.
About the type I don't think i would have problem, because when creating the
trpcClient
in NextJs, i use the AppRouter
type from my internal package where i declare the appRouter and the procedures:
if i understand the flow correctly, the image describe the flow. so i don't have type problem
The Red part is what i'm trying to solve
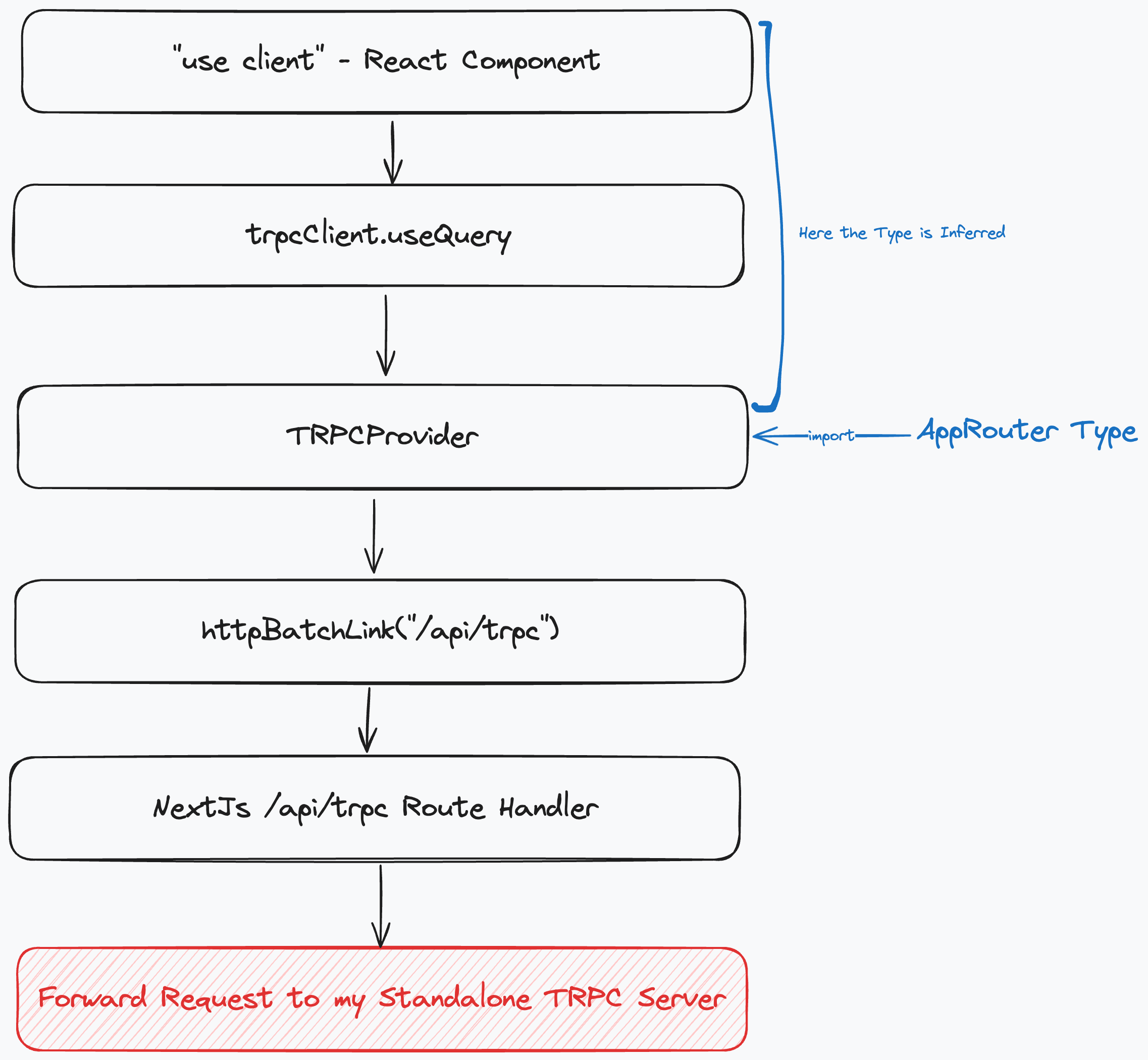
in the same concept when i call trpc from my server component it work with the type infer:
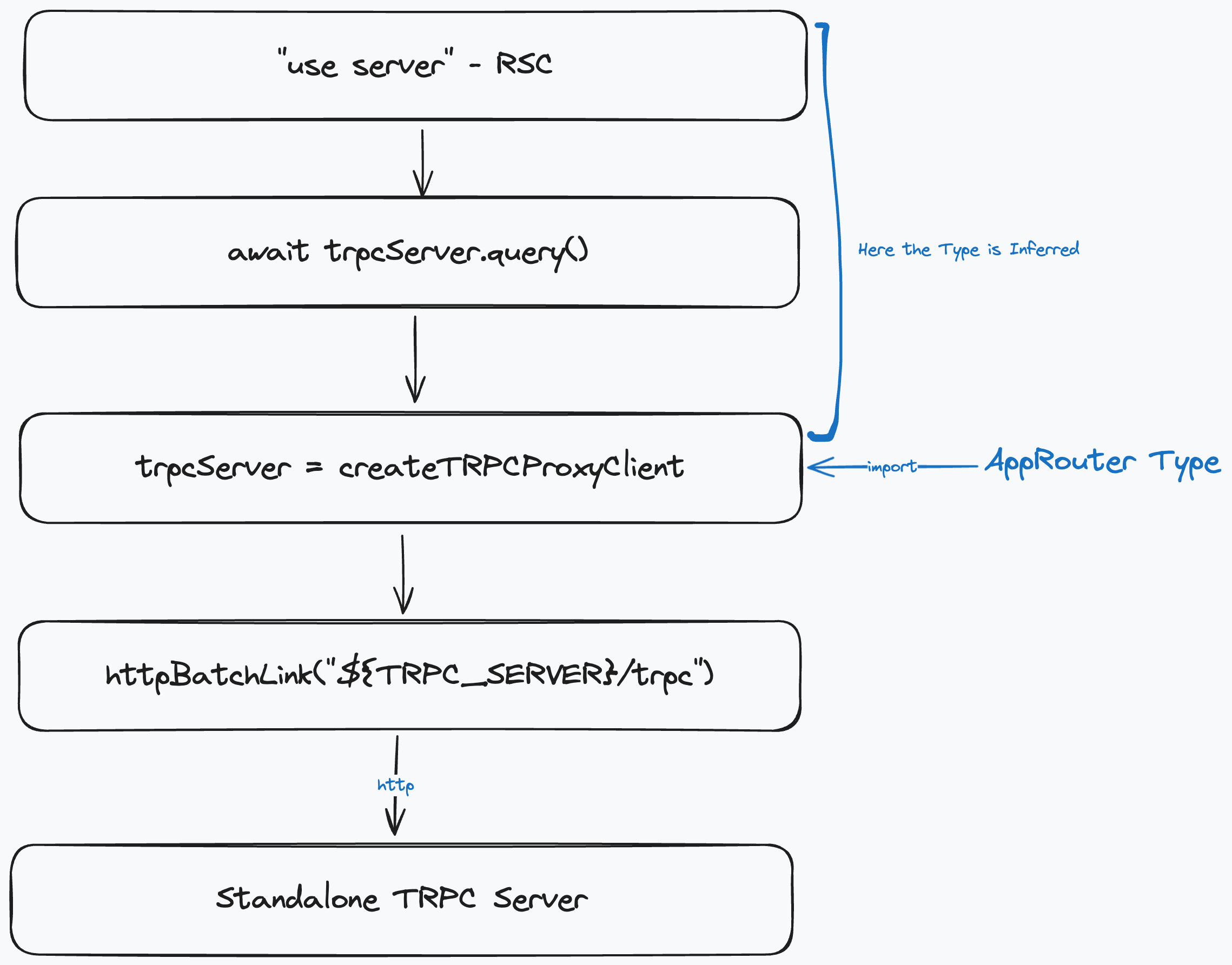
for now i found doing this is working, but i really don't now when it will bite me back:
@Poonda curious, why not talk to the trpc server directly?
react-native <-> trpc
next.js <-> trpc
security, to not expose my TRPC server to the client side
(I personally don't understand fully the why, but that's the task I got, I've tried to argue diffrently, but I'm not the Team Lead :D)
wondering if you were able to solve this issue.
@david I also have this case, the api was developed separately and is much older than our nextjs app.
it’s been a while since i’ve worked on our tRPC project, happy to try and help if i can again @Ahmed Eid is the case that you’re basically just trying to have the request proxied through an older api and are asking about recommended practices?
yes exactly.
similar to this.
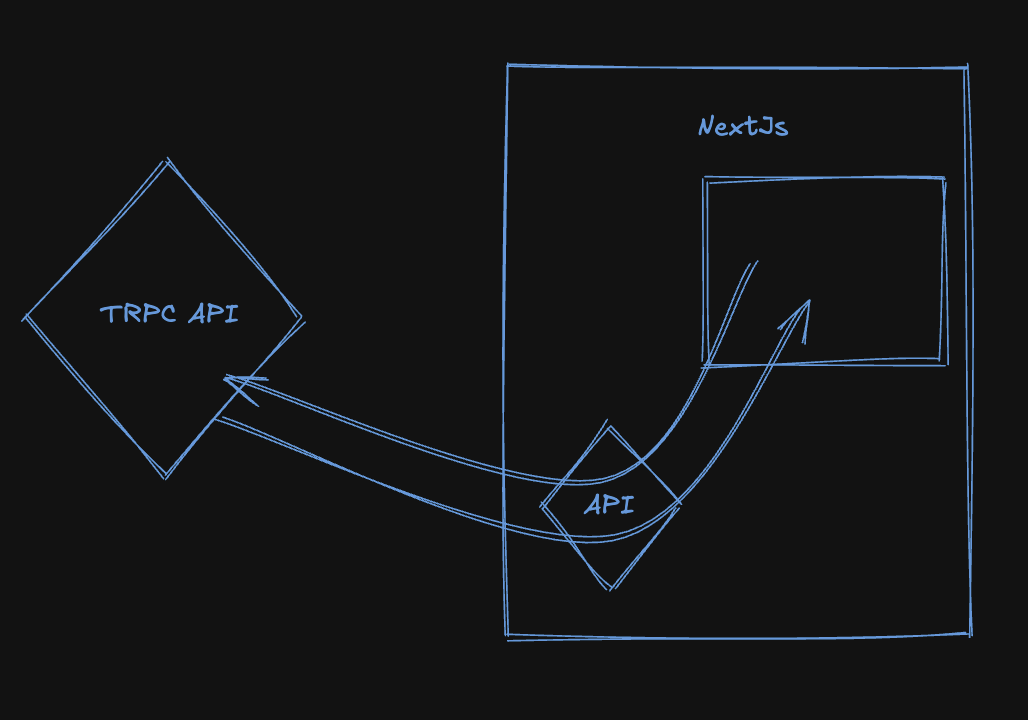
I currently have my nextjs app calling TRPC API directly which is working fine, however I have been facing issues with cross site cookies.
when queries / mutations are ran on the client.
someone’s welcome to add if they want,
personally i mean in some applications all things considered if this is the route you have to go through. transparent proxying the request via your next js app as its acting as a gateway now is probably fine?
i’m imagining the reason for why you need to speak to the next api first is probably some security/auth setup in your next app, so outside of added round trip latency and assuming you’re not modifying the request body on the return (so that tRPC and react query knows how the data is saved) i don’t see any problems?
okay well hold on a sec, before going all in on proxying the request through next js you might want to look into trying to fix the CORS issue first no? otherwise you are trying to resolve a symptom than the actual issue? can try and help with that if i can
could you please explain what you mean, I have not CORS issues. the url is white listed on my TRPC service.
You’re saying you’re having issues with cross-site cookies, right? If the goal is to have tRPC communicating with your Next.js client app, can you clarify what exactly isn’t working? Are cookies not being sent at all, or are they not persisting across requests?
yeah that's the main issue, I am not able to set / send cookies from frontend to my TRPC service.
any chance you can send over some of the code you’re using to setup the cookie? similarly, in your react query context/config are you able to show some of that code as well? like how the trpc.createClient looks?
I think if I am able to proxy those requests, then I could transform data to / from my service easily.
so mainly I am setting cookies in my middleware like so.
I tried adding an origin, however it didn't work.
sure it could work, but it could also be unnecessary if the root cause is cors/a credentials misconfiguration - you’re sort of masking the issue rather than fixing it directly in this case..
if you’d be up to check how the cookies are setup and your configs are working it could be useful before going down the proxy route. just my feeling
ahmed can you show on your next client how the trpc config is setup by any chance?
The main thing I wanted to check was whether you had credentials: "include", which you do—so that’s all good.
just checking—on your tRPC server, how are you configuring your cookie policy? Are you setting SameSite=None if it’s a cross-origin request? If the frontend and backend are on different origins, browsers require these settings for cookies to work iirc. also, are you setting Access-Control-Allow-Credentials: true in your CORS config? (in express it’s just credentials:true i think)
yeah. I am using hono.
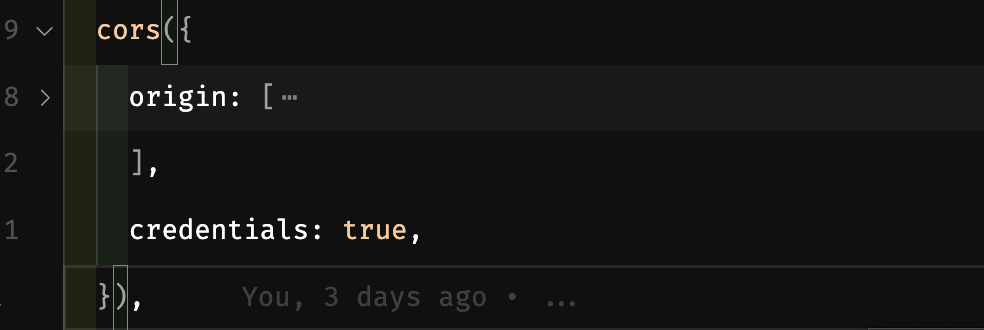
hm, i’m not 100% sure but i think it could be to do with the way you’ve setup your cookies, it might need to be modified with samesite: “none” and secure: true in the third argument of the response.cookies.set
think you might have concerns about CSRF but you could try it at the very least to see if you stop having issues by doing that.. something like
i think you can also setup which domains the cookie can run with? so that might work? https://nextjs.org/docs/app/api-reference/functions/cookies
alternatively yeah, proxying the request via your next js app might be another direction. just giving you the heads up that this might be what fixes the issue for you via the cookies
Functions: cookies | Next.js
API Reference for the cookies function.